1. 概要
今回はPrismaを使ってSQLiteにデータを登録しながら抽出まで行う内容になります。
対象としては開発を1年程やってて自分で最初から開発してみたい方になります。そのため細かい用語などの説明はしません。
2. Prismaとは
- ORM for Node.js & Typescript
Node.jsとTypeScriptで利用できるORM(Object Relational Mapping)のようです。
Prisma | Next-generation ORM for Node.js & TypeScript
3. nodeのインストール
こちらを参考
ここからは公式サイトの手順に従い、やってみます。
4. プロジェクトを作成
mkdir hello-prisma
cd hello-prisma
5. 必要なライブラリをインストール
npm init -y
npm install typescript ts-node @types/node --save-dev
npx tsc --init
npm install prisma --save-dev
npx prisma init --datasource-provider sqlite
6. modelを定義
※prisma/schema.prisma
// This is your Prisma schema file,
// learn more about it in the docs: https://pris.ly/d/prisma-schema
generator client {
provider = "prisma-client-js"
}
datasource db {
provider = "sqlite"
url = env("DATABASE_URL")
}
model User {
id Int @id @default(autoincrement())
email String @unique
name String?
posts Post[]
}
model Post {
id Int @id @default(autoincrement())
title String
content String?
published Boolean @default(false)
author User @relation(fields: [authorId], references: [id])
authorId Int
}
- model「User」「Post」を追加
7. DBを作成
npx prisma migrate dev --name init
Environment variables loaded from .env
Prisma schema loaded from prisma/schema.prisma
Datasource "db": SQLite database "dev.db" at "file:./dev.db"
SQLite database dev.db created at file:./dev.db
Applying migration `20230709011323_init`
The following migration(s) have been created and applied from new schema changes:
migrations/
└─ 20230709011323_init/
└─ migration.sql
Your database is now in sync with your schema.
Running generate... (Use --skip-generate to skip the generators)
added 2 packages, and audited 24 packages in 8s
found 0 vulnerabilities
✔ Generated Prisma Client (4.16.2 | library) to ./node_modules/@prisma/client in 60ms
※.env
# Environment variables declared in this file are automatically made available to Prisma.
# See the documentation for more detail: https://pris.ly/d/prisma-schema#accessing-environment-variables-from-the-schema
# Prisma supports the native connection string format for PostgreSQL, MySQL, SQLite, SQL Server, MongoDB and CockroachDB.
# See the documentation for all the connection string options: https://pris.ly/d/connection-strings
DATABASE_URL="file:./dev.db"
8. Userデータを登録
※src/create-user.ts
import { PrismaClient } from '@prisma/client'
const prisma = new PrismaClient()
async function main() {
const user = await prisma.user.create({
data: {
name: 'Alice',
email: 'alice@prisma.io',
},
})
console.log(user)
}
main()
.then(async () => {
await prisma.$disconnect()
})
.catch(async (e) => {
console.error(e)
await prisma.$disconnect()
process.exit(1)
})
実行
npx ts-node src/create-user.ts
{ id: 1, email: 'alice@prisma.io', name: 'Alice' }
9. Userデータを取得
※src/select-user.ts
import { PrismaClient } from '@prisma/client'
const prisma = new PrismaClient()
async function main() {
const users = await prisma.user.findMany()
console.log(users)
}
main()
.then(async () => {
await prisma.$disconnect()
})
.catch(async (e) => {
console.error(e)
await prisma.$disconnect()
process.exit(1)
})
実行
npx ts-node src/select-user.ts
[ { id: 1, email: 'alice@prisma.io', name: 'Alice' } ]
10. Postデータを登録
※src/create-post.ts
import { PrismaClient } from '@prisma/client'
const prisma = new PrismaClient()
async function main() {
const user = await prisma.user.create({
data: {
name: 'Bob',
email: 'bob@prisma.io',
posts: {
create: {
title: 'Hello World',
},
},
},
})
console.log(user)
}
main()
.then(async () => {
await prisma.$disconnect()
})
.catch(async (e) => {
console.error(e)
await prisma.$disconnect()
process.exit(1)
})
実行
npx ts-node src/create-post.ts
{ id: 2, email: 'bob@prisma.io', name: 'Bob' }
11. Postデータを取得
※src/select-post.ts
import { PrismaClient } from '@prisma/client'
const prisma = new PrismaClient()
async function main() {
const usersWithPosts = await prisma.user.findMany({
include: {
posts: true,
},
})
console.dir(usersWithPosts, { depth: null })
}
main()
.then(async () => {
await prisma.$disconnect()
})
.catch(async (e) => {
console.error(e)
await prisma.$disconnect()
process.exit(1)
})
実行
npx ts-node src/select-post.ts
[
{ id: 1, email: 'alice@prisma.io', name: 'Alice', posts: [] },
{
id: 2,
email: 'bob@prisma.io',
name: 'Bob',
posts: [
{
id: 1,
title: 'Hello World',
content: null,
published: false,
authorId: 2
}
]
}
]
12. Prisma Studioで確認
※Built-in GUIでデータを確認
npx prisma studio
Environment variables loaded from .env
Prisma schema loaded from prisma/schema.prisma
Prisma Studio is up on http://localhost:5555
- http://localhost:5555
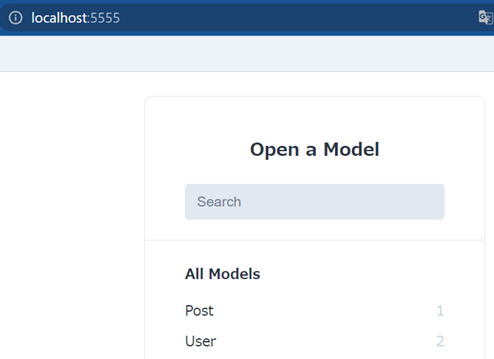
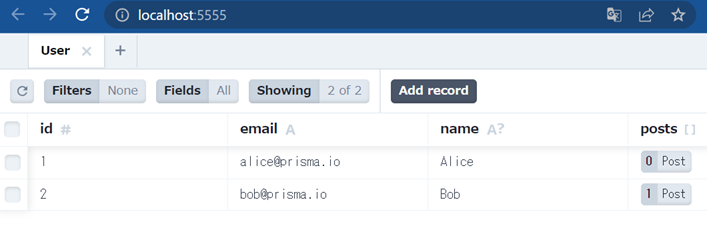

13. ディレクトリの構造
.
├── .env
├── node_modules
├── package-lock.json
├── package.json
├── prisma
│ ├── dev.db
│ ├── migrations
│ │ ├── 20230709011323_init
│ │ │ └── migration.sql
│ │ └── migration_lock.toml
│ └── schema.prisma
├── src
│ ├── create-post.ts
│ ├── create-user.ts
│ ├── select-post.ts
│ └── select-user.ts
└── tsconfig.json
14. 備考
今回は公式サイトの手順に従い、Prismaを使ってデータを操作するシンプルな内容でした。
15. 参考
投稿者プロフィール

-
開発好きなシステムエンジニアです。
卓球にハマってます。