1. 概要
前回はRedux Thunksを使いAPI呼び出しの非同期処理についてでした。今回はMetadata(Head, Title)やScript、Parametersの使い方についてです。
対象としては開発を1年程やってて自分で最初から開発してみたい方になります。そのため細かい用語などの説明はしません。
2. nodeのインストール
こちらを参考
3. プロジェクトを作成
こちらを参考
4. 必要なライブラリをインストール
こちらを参考
5. ソースコード
※前回より差分のみを記載
5-1-1. public/js/script.js
alert("Run JS on each page");
5-1-2. src/app/nextjs/nextjs01/child/metadata.ts
import { Metadata } from "next";
export const metadata: Metadata = {
title: "New title",
description: "Usage of Metadata",
robots: {
index: false,
follow: true,
nocache: true,
googleBot: {
index: true,
follow: false,
noimageindex: true,
"max-video-preview": -1,
"max-image-preview": "large",
"max-snippet": -1,
},
},
};
5-1-3. src/app/nextjs/nextjs01/child/client-page.tsx
"use client";
import Script from "next/script";
import { useSearchParams } from "next/navigation";
import scss from "../page.module.scss";
import GoBack from "@/lib/components/go-back";
const callMe = () => {
alert("The external JS is called.");
};
const ClientPage = () => {
const searchParams = useSearchParams();
const param: string | null = searchParams.get("param");
return (
<>
<div className={scss.component}>
<GoBack />
<br />
<br />
<ul>
<li>Metadata</li>
<ul>
<li>Title</li>
</ul>
<li>Script</li>
<ul>
<li>External JavaScript</li>
</ul>
<li>Parameters</li>
<ul>
<li>Param: {param}</li>
</ul>
</ul>
<br />
</div>
<Script
src="http://localhost:3000/js/script.js"
strategy="afterInteractive"
onLoad={callMe}
/>
</>
);
};
export default ClientPage;
5-1-4. src/app/nextjs/nextjs01/child/page.tsx
import ClientPage from "./client-page";
import { metadata } from "./metadata";
export { metadata };
const Child = () => {
return (
<>
<ClientPage />
</>
);
};
export default Child;
5-1-5. src/app/nextjs/nextjs01/page.module.scss
.component {
color: blue;
& ul {
margin-left: 20px;
& li {
list-style: disc;
}
}
}
5-1-6. src/app/nextjs/nextjs01/page.tsx
"use client";
import Link from "next/link";
import { useRouter } from "next/navigation";
import { Button } from "@mui/material";
import scss from "./page.module.scss";
import GoBack from "@/lib/components/go-back";
const Nextjs01 = () => {
const router = useRouter();
return (
<div className={scss.component}>
<GoBack />
<br />
<br />
<ul>
<li>Navigation</li>
<ul>
<li>
<Link
href={{ pathname: "./nextjs01/child", query: { param: "link" } }}
>
Link
</Link>
</li>
<li>
<Button
variant="contained"
size="medium"
color="primary"
onClick={() => router.push("./nextjs01/child?param=button")}
>
Button (useRouter)
</Button>
</li>
</ul>
</ul>
<br />
</div>
);
};
export default Nextjs01;
5-1-7. src/app/nextjs/page.module.scss
.components {
color: blue;
& ul {
margin-left: 20px;
& li {
list-style: disc;
}
}
}
5-1-8. src/app/nextjs/page.tsx
"use client";
import React from "react";
import { Link } from "@mui/material";
import scss from "./page.module.scss";
const Nextjs = () => {
return (
<div className={scss.components}>
<ul>
<li>
<Link href="/nextjs/nextjs01" underline="hover">
Nextjs01
</Link>
</li>
</ul>
</div>
);
};
export default Nextjs;
5-1-9. src/lib/common/go-back.tsx
"use client";
import { useRouter } from "next/navigation";
import { Button } from "@mui/material";
const GoBack = () => {
const router = useRouter();
return (
<Button
variant="outlined"
size="small"
color="secondary"
onClick={() => router.back()}
>
Go back
</Button>
);
};
export default GoBack;
5-1-10. src/lib/common/sidebar-links.tsx
import HomeIcon from "@mui/icons-material/Home";
import AdjustIcon from "@mui/icons-material/Adjust";
import { SidebarLinkType } from "./definitions";
const sidebarHome: SidebarLinkType = {
label: "Home",
path: "/",
icon: <HomeIcon />,
targetSegment: null,
};
const sidebarComponents: SidebarLinkType = {
label: "Components",
path: "/components",
icon: <AdjustIcon />,
targetSegment: "components",
};
const sidebarEvents: SidebarLinkType = {
label: "Events",
path: "/events",
icon: <AdjustIcon />,
targetSegment: "events",
};
const sidebarHooks: SidebarLinkType = {
label: "Hooks",
path: "/hooks",
icon: <AdjustIcon />,
targetSegment: "hooks",
};
const sidebarRedux: SidebarLinkType = {
label: "Redux",
path: "/redux",
icon: <AdjustIcon />,
targetSegment: "redux",
};
const sidebarNextjs: SidebarLinkType = {
label: "Nextjs",
path: "/nextjs",
icon: <AdjustIcon />,
targetSegment: "nextjs",
};
export const sidebarLinks: SidebarLinkType[] = [
sidebarHome,
sidebarComponents,
sidebarEvents,
sidebarHooks,
sidebarRedux,
sidebarNextjs,
];
6. サーバーを起動
npm run dev
7. ブラウザで確認
- http://localhost:3000
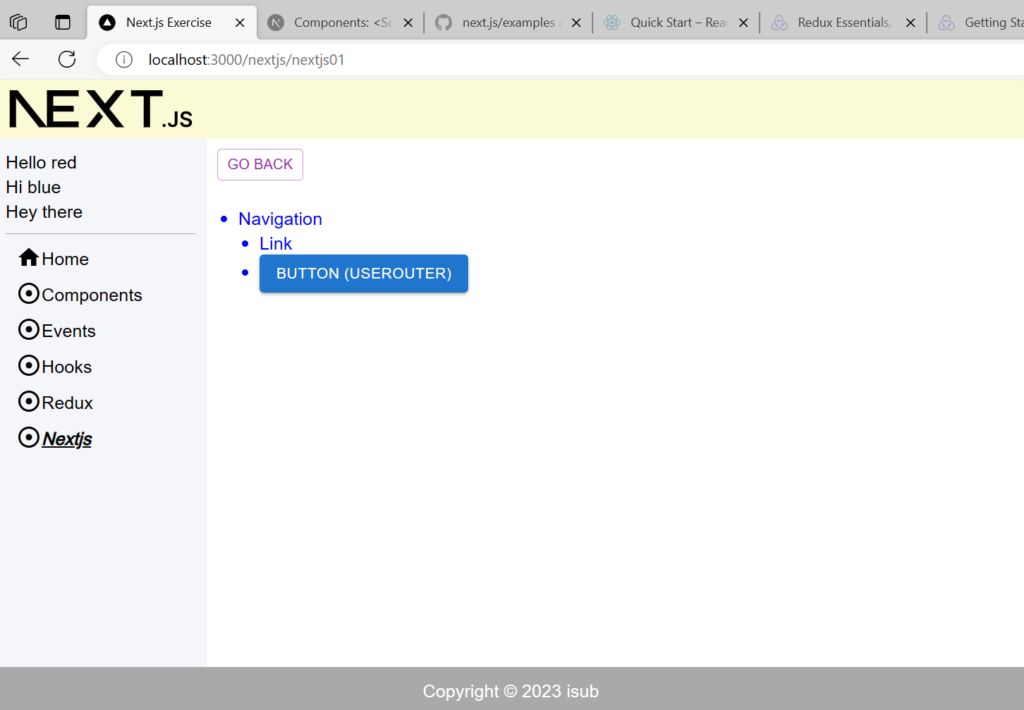
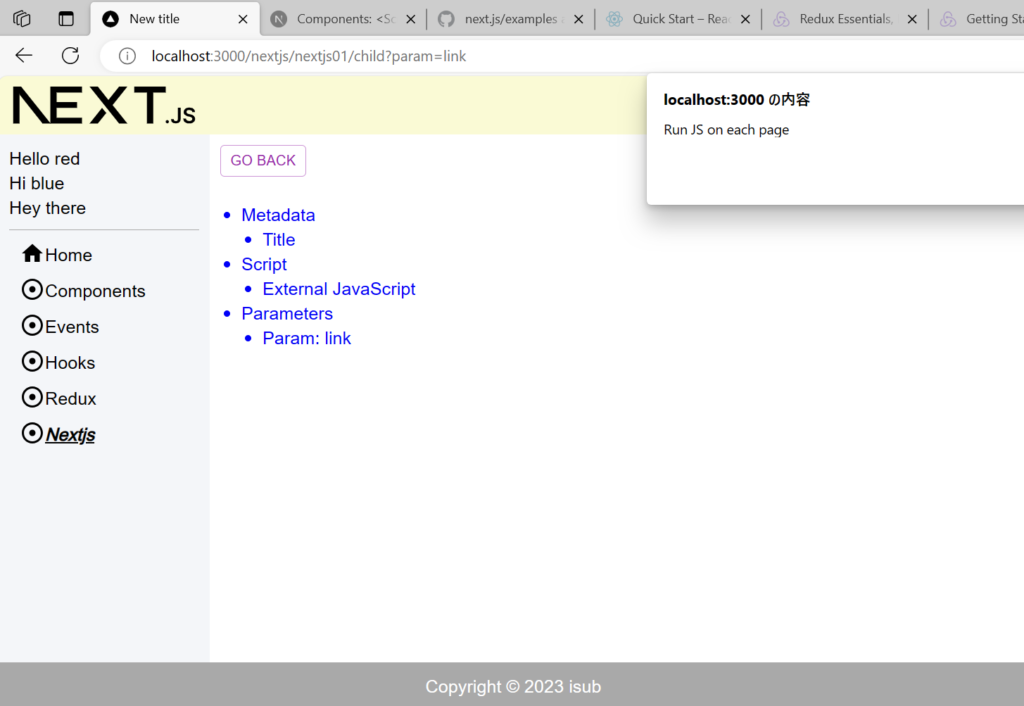
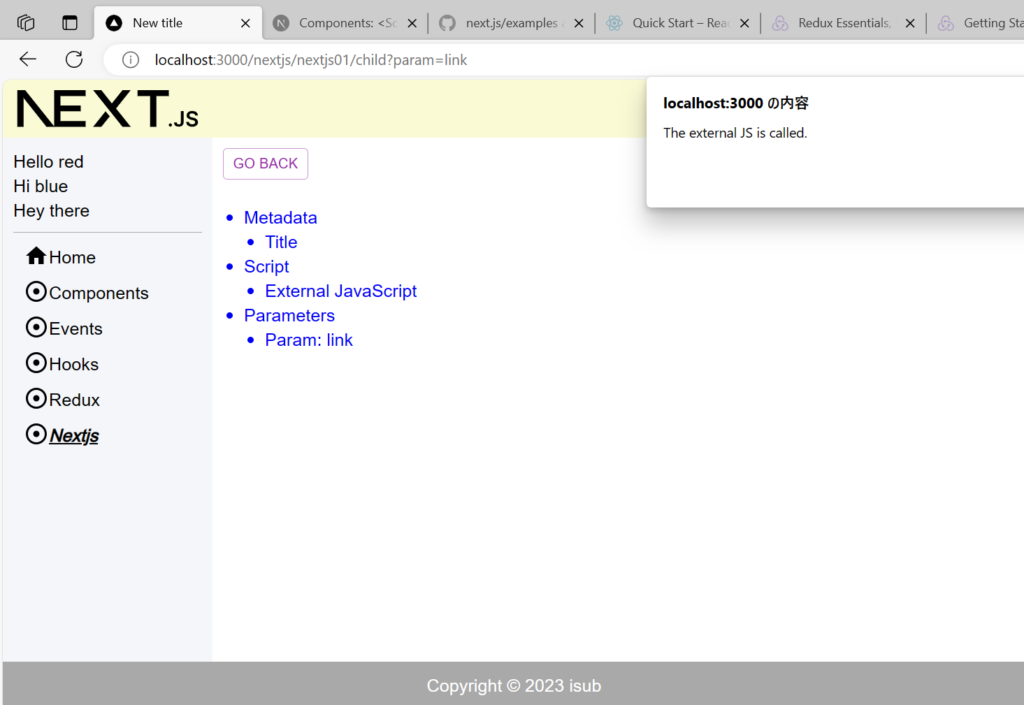
8. ディレクトリの構造
.
├── README.md
├── next-env.d.ts
├── next.config.js
├── package-lock.json
├── package.json
├── postcss.config.js
├── public
│ ├── js
│ │ └── script.js
│ ├── next.svg
│ └── vercel.svg
├── src
│ ├── app
│ │ ├── components
│ │ │ ├── component01
│ │ │ │ ├── page.module.scss
│ │ │ │ └── page.tsx
│ │ │ ├── component02
│ │ │ │ ├── page.module.scss
│ │ │ │ └── page.tsx
│ │ │ ├── component03
│ │ │ │ ├── page.module.scss
│ │ │ │ └── page.tsx
│ │ │ ├── component04
│ │ │ │ ├── checkbox-demo.tsx
│ │ │ │ ├── page.module.scss
│ │ │ │ ├── page.tsx
│ │ │ │ ├── radio-demo.tsx
│ │ │ │ └── select-demo.tsx
│ │ │ ├── page.module.scss
│ │ │ └── page.tsx
│ │ ├── events
│ │ │ ├── event01
│ │ │ │ ├── page.module.scss
│ │ │ │ └── page.tsx
│ │ │ ├── page.module.scss
│ │ │ └── page.tsx
│ │ ├── favicon.ico
│ │ ├── globals.css
│ │ ├── globals.scss
│ │ ├── hooks
│ │ │ ├── hook01
│ │ │ │ ├── page.module.scss
│ │ │ │ └── page.tsx
│ │ │ ├── hook02
│ │ │ │ ├── page.module.scss
│ │ │ │ └── page.tsx
│ │ │ ├── hook03
│ │ │ │ ├── child.tsx
│ │ │ │ ├── counter-provider.tsx
│ │ │ │ ├── grandchild.tsx
│ │ │ │ ├── myself.tsx
│ │ │ │ ├── page.module.scss
│ │ │ │ └── page.tsx
│ │ │ ├── hook04
│ │ │ │ ├── page.module.scss
│ │ │ │ └── page.tsx
│ │ │ ├── hook05
│ │ │ │ ├── child.tsx
│ │ │ │ ├── counter-provider.tsx
│ │ │ │ ├── grandchild.tsx
│ │ │ │ ├── myself.tsx
│ │ │ │ ├── page.module.scss
│ │ │ │ └── page.tsx
│ │ │ ├── hook06
│ │ │ │ ├── child.tsx
│ │ │ │ ├── page.module.scss
│ │ │ │ ├── page.tsx
│ │ │ │ ├── play-provider.tsx
│ │ │ │ ├── text-box.tsx
│ │ │ │ └── video-player.tsx
│ │ │ ├── page.module.scss
│ │ │ └── page.tsx
│ │ ├── layout.module.scss
│ │ ├── layout.tsx
│ │ ├── nextjs
│ │ │ ├── nextjs01
│ │ │ │ ├── child
│ │ │ │ │ ├── client-page.tsx
│ │ │ │ │ ├── metadata.ts
│ │ │ │ │ └── page.tsx
│ │ │ │ ├── page.module.scss
│ │ │ │ └── page.tsx
│ │ │ ├── page.module.scss
│ │ │ └── page.tsx
│ │ ├── page.module.scss
│ │ ├── page.tsx
│ │ └── redux
│ │ ├── page.module.scss
│ │ ├── page.tsx
│ │ ├── redux01
│ │ │ ├── child.tsx
│ │ │ ├── counter-slice.ts
│ │ │ ├── grandchild.tsx
│ │ │ ├── hooks.ts
│ │ │ ├── myself.tsx
│ │ │ ├── page.module.scss
│ │ │ ├── page.tsx
│ │ │ └── store.ts
│ │ └── redux02
│ │ ├── child.tsx
│ │ ├── hooks.ts
│ │ ├── image-box.tsx
│ │ ├── image-slice.ts
│ │ ├── page.module.scss
│ │ ├── page.tsx
│ │ ├── store.ts
│ │ ├── text-box.tsx
│ │ └── text-slice.ts
│ ├── lib
│ │ ├── common
│ │ │ ├── definitions.ts
│ │ │ └── sidebar-links.tsx
│ │ ├── components
│ │ │ ├── alert-snackbar.tsx
│ │ │ ├── go-back.tsx
│ │ │ └── spacer.tsx
│ │ ├── footer.tsx
│ │ ├── header.tsx
│ │ ├── sidebar.tsx
│ │ └── utils
│ │ └── util.ts
│ └── scss
│ └── common
│ ├── _index.scss
│ ├── _mixin.scss
│ ├── _mq.scss
│ └── _variables.scss
├── tailwind.config.ts
└── tsconfig.json
30 directories, 100 files
9. 備考
今回はMetadata(Head, Title)やScript、Parametersの使い方についてでした。
10. 参考
- Docs | Next.js (nextjs.org)
- Quick Start – React
- Getting Started with Redux | Redux
- Getting Started with React Redux | React Redux (react-redux.js.org)
- Material UI: React components based on Material Design (mui.com)
投稿者プロフィール

-
開発好きなシステムエンジニアです。
卓球にハマってます。
最新の投稿
【AWS】2024年4月27日【AWS】Amazon DynamoDBを使ってみる(CLI-API)
【AWS】2024年4月25日【AWS】Amazon DynamoDBを使ってみる(Management Console)
【AWS】2024年4月22日【AWS】API Gatewayを使ってみる
【AWS】2024年4月18日【AWS】Lambdaを使ってみる