1. 概要
前回はMetadata(Head, Title)やScript、Parametersの使い方についてでした。今回はDynamic Routesの使い方についてです。
対象としては開発を1年程やってて自分で最初から開発してみたい方になります。そのため細かい用語などの説明はしません。
2. nodeのインストール
こちらを参考
3. プロジェクトを作成
こちらを参考
4. 必要なライブラリをインストール
こちらを参考
5. ソースコード
※前回より差分のみを記載
5-1-1. src/app/nextjs/nextjs02/shop/[id]
shop/[id]ディレクトリを作成
5-1-2. src/app/nextjs/nextjs02/shop/[id]/page.tsx
"use client";
import { useSearchParams } from "next/navigation";
import scss from "../../page.module.scss";
import GoBack from "@/lib/components/go-back";
const Id = ({ params }: { params: { id: string } }) => {
const searchParams = useSearchParams();
const param: string | null = searchParams.get("param");
return (
<div className={scss.component}>
<GoBack />
<br />
<br />
<ul>
<li>Parameters</li>
<ul>
<li>{param}</li>
</ul>
<li>shop</li>
<ul>
<li>{params.id}</li>
</ul>
</ul>
</div>
);
};
export default Id;
5-1-3. src/app/nextjs/nextjs02/[…slug]
[...slug]ディレクトリを作成
5-1-4. src/app/nextjs/nextjs02/[…slug]/page.tsx
"use client";
import { useSearchParams } from "next/navigation";
import scss from "../page.module.scss";
import GoBack from "@/lib/components/go-back";
const Slug = ({ params }: { params: { slug: string } }) => {
const liElements = Object.entries(params.slug).map(([idx, e]) => (
<li key={idx}>{`${parseInt(idx) + 1} ⇒ ${e}`}</li>
));
const searchParams = useSearchParams();
const param: string | null = searchParams.get("param");
return (
<div className={scss.component}>
<GoBack />
<br />
<br />
<ul>
<li>Parameters</li>
<ul>
<li>{param}</li>
</ul>
<li>Hierachy</li>
<ul>{liElements}</ul>
</ul>
</div>
);
};
export default Slug;
5-1-5. src/app/nextjs/nextjs02/page.module.scss
.component {
color: blue;
& ul {
margin-left: 20px;
& li {
list-style: disc;
}
}
}
5-1-6. src/app/nextjs/nextjs02/page.tsx
import Link from "next/link";
import scss from "./page.module.scss";
import GoBack from "@/lib/components/go-back";
const Nextjs02 = () => {
return (
<div className={scss.component}>
<GoBack />
<br />
<br />
<ul>
<li>Dynamic Routes</li>
<ul>
<li>Only one segment</li>
<ul>
<li>
<Link
href={{
pathname: "./nextjs02/shop/arikashop",
query: { param: "link" },
}}
>
shop/[id] -> shop/arikashop
</Link>
</li>
</ul>
<li>All segments</li>
<ul>
<li>
<Link
href={{ pathname: "./nextjs02/blog", query: { param: "link" } }}
>
[...slug] -> blog
</Link>
</li>
<li>
<Link
href={{
pathname: "./nextjs02/blog/isub",
query: { param: "link" },
}}
>
[...slug] -> blog/isub
</Link>
</li>
<li>
<Link
href={{
pathname: "./nextjs02/blog/isub/about",
query: { param: "link" },
}}
>
[...slug] -> blog/isub/about
</Link>
</li>
</ul>
</ul>
</ul>
<br />
</div>
);
};
export default Nextjs02;
5-1-7. src/app/nextjs/page.module.scss
.components {
color: blue;
& ul {
margin-left: 20px;
& li {
list-style: disc;
}
}
}
5-1-8. src/app/nextjs/page.tsx
"use client";
import React from "react";
import { Link } from "@mui/material";
import scss from "./page.module.scss";
const Nextjs = () => {
return (
<div className={scss.components}>
<ul>
<li>
<Link href="/nextjs/nextjs01" underline="hover">
Nextjs01
</Link>
</li>
<li>
<Link href="/nextjs/nextjs02" underline="hover">
Nextjs02
</Link>
</li>
</ul>
</div>
);
};
export default Nextjs;
6. サーバーを起動
npm run dev
7. ブラウザで確認
- http://localhost:3000
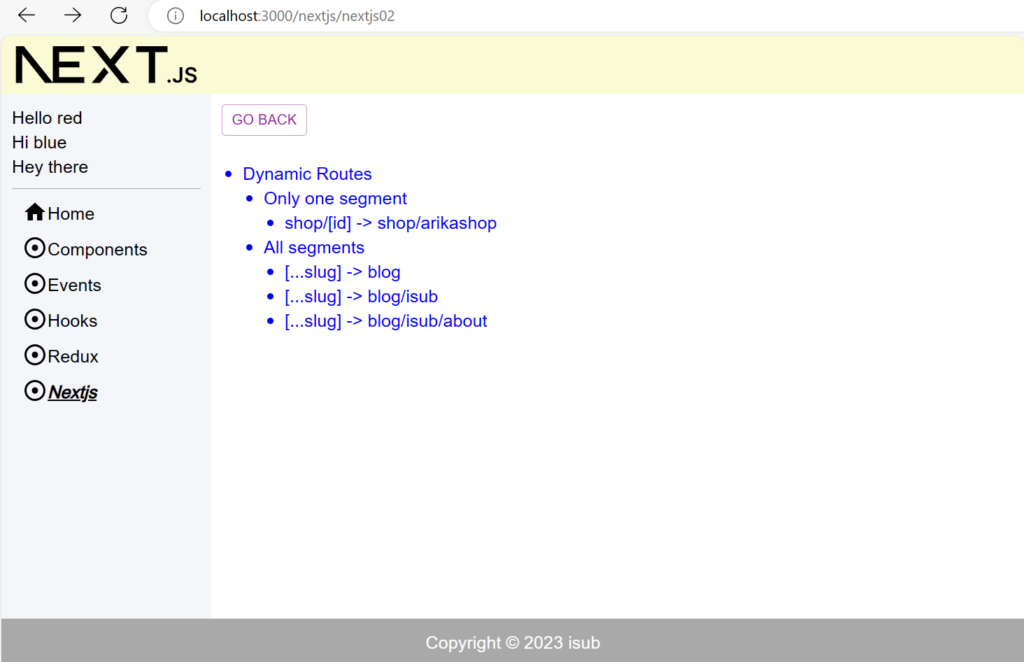
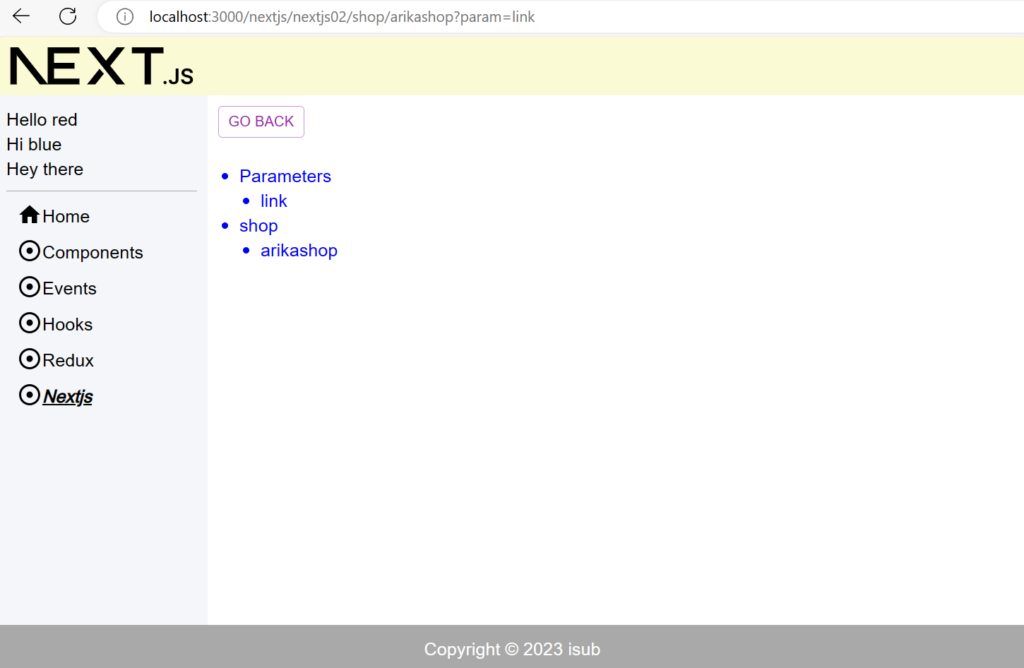
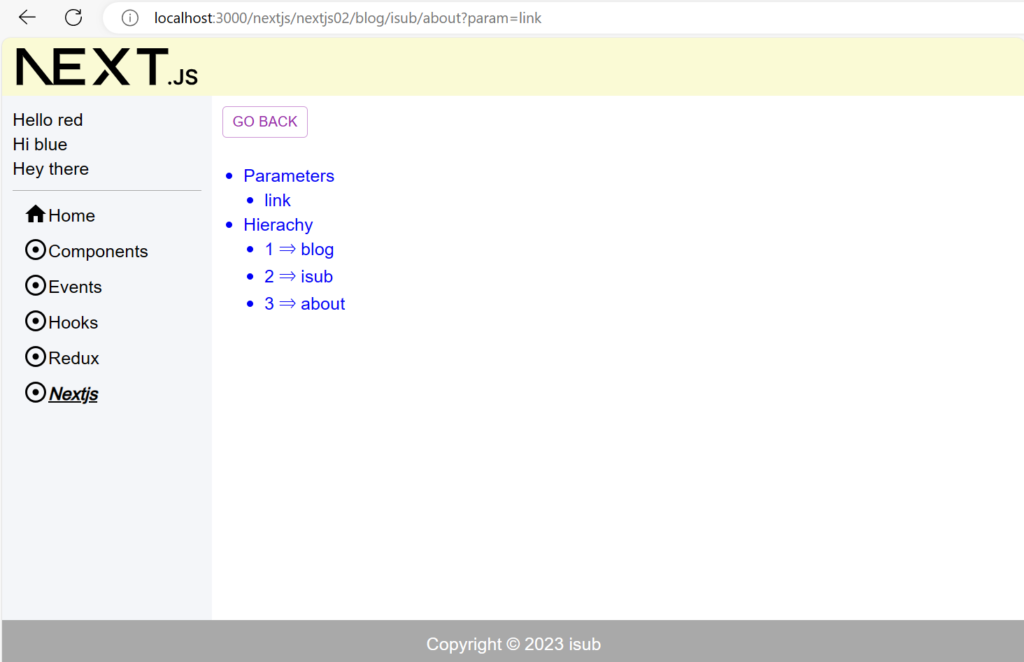
8. ディレクトリの構造
.
├── README.md
├── next-env.d.ts
├── next.config.js
├── package-lock.json
├── package.json
├── postcss.config.js
├── public
│ ├── js
│ │ └── script.js
│ ├── next.svg
│ └── vercel.svg
├── src
│ ├── app
│ │ ├── components
│ │ │ ├── component01
│ │ │ │ ├── page.module.scss
│ │ │ │ └── page.tsx
│ │ │ ├── component02
│ │ │ │ ├── page.module.scss
│ │ │ │ └── page.tsx
│ │ │ ├── component03
│ │ │ │ ├── page.module.scss
│ │ │ │ └── page.tsx
│ │ │ ├── component04
│ │ │ │ ├── checkbox-demo.tsx
│ │ │ │ ├── page.module.scss
│ │ │ │ ├── page.tsx
│ │ │ │ ├── radio-demo.tsx
│ │ │ │ └── select-demo.tsx
│ │ │ ├── page.module.scss
│ │ │ └── page.tsx
│ │ ├── events
│ │ │ ├── event01
│ │ │ │ ├── page.module.scss
│ │ │ │ └── page.tsx
│ │ │ ├── page.module.scss
│ │ │ └── page.tsx
│ │ ├── favicon.ico
│ │ ├── globals.css
│ │ ├── globals.scss
│ │ ├── hooks
│ │ │ ├── hook01
│ │ │ │ ├── page.module.scss
│ │ │ │ └── page.tsx
│ │ │ ├── hook02
│ │ │ │ ├── page.module.scss
│ │ │ │ └── page.tsx
│ │ │ ├── hook03
│ │ │ │ ├── child.tsx
│ │ │ │ ├── counter-provider.tsx
│ │ │ │ ├── grandchild.tsx
│ │ │ │ ├── myself.tsx
│ │ │ │ ├── page.module.scss
│ │ │ │ └── page.tsx
│ │ │ ├── hook04
│ │ │ │ ├── page.module.scss
│ │ │ │ └── page.tsx
│ │ │ ├── hook05
│ │ │ │ ├── child.tsx
│ │ │ │ ├── counter-provider.tsx
│ │ │ │ ├── grandchild.tsx
│ │ │ │ ├── myself.tsx
│ │ │ │ ├── page.module.scss
│ │ │ │ └── page.tsx
│ │ │ ├── hook06
│ │ │ │ ├── child.tsx
│ │ │ │ ├── page.module.scss
│ │ │ │ ├── page.tsx
│ │ │ │ ├── play-provider.tsx
│ │ │ │ ├── text-box.tsx
│ │ │ │ └── video-player.tsx
│ │ │ ├── page.module.scss
│ │ │ └── page.tsx
│ │ ├── layout.module.scss
│ │ ├── layout.tsx
│ │ ├── nextjs
│ │ │ ├── nextjs01
│ │ │ │ ├── child
│ │ │ │ │ ├── client-page.tsx
│ │ │ │ │ ├── metadata.ts
│ │ │ │ │ └── page.tsx
│ │ │ │ ├── page.module.scss
│ │ │ │ └── page.tsx
│ │ │ ├── nextjs02
│ │ │ │ ├── [...slug]
│ │ │ │ │ └── page.tsx
│ │ │ │ ├── page.module.scss
│ │ │ │ ├── page.tsx
│ │ │ │ └── shop
│ │ │ │ └── [id]
│ │ │ │ └── page.tsx
│ │ │ ├── page.module.scss
│ │ │ └── page.tsx
│ │ ├── page.module.scss
│ │ ├── page.tsx
│ │ └── redux
│ │ ├── page.module.scss
│ │ ├── page.tsx
│ │ ├── redux01
│ │ │ ├── child.tsx
│ │ │ ├── counter-slice.ts
│ │ │ ├── grandchild.tsx
│ │ │ ├── hooks.ts
│ │ │ ├── myself.tsx
│ │ │ ├── page.module.scss
│ │ │ ├── page.tsx
│ │ │ └── store.ts
│ │ └── redux02
│ │ ├── child.tsx
│ │ ├── hooks.ts
│ │ ├── image-box.tsx
│ │ ├── image-slice.ts
│ │ ├── page.module.scss
│ │ ├── page.tsx
│ │ ├── store.ts
│ │ ├── text-box.tsx
│ │ └── text-slice.ts
│ ├── lib
│ │ ├── common
│ │ │ ├── definitions.ts
│ │ │ └── sidebar-links.tsx
│ │ ├── components
│ │ │ ├── alert-snackbar.tsx
│ │ │ ├── go-back.tsx
│ │ │ └── spacer.tsx
│ │ ├── footer.tsx
│ │ ├── header.tsx
│ │ ├── sidebar.tsx
│ │ └── utils
│ │ └── util.ts
│ └── scss
│ └── common
│ ├── _index.scss
│ ├── _mixin.scss
│ ├── _mq.scss
│ └── _variables.scss
├── tailwind.config.ts
└── tsconfig.json
34 directories, 104 files
9. 備考
今回はDynamic Routesの使い方についてでした。
10. 参考
- Docs | Next.js (nextjs.org)
- Quick Start – React
- Getting Started with Redux | Redux
- Getting Started with React Redux | React Redux (react-redux.js.org)
- Material UI: React components based on Material Design (mui.com)
投稿者プロフィール

-
開発好きなシステムエンジニアです。
卓球にハマってます。
最新の投稿
【AWS】2024年4月27日【AWS】Amazon DynamoDBを使ってみる(CLI-API)
【AWS】2024年4月25日【AWS】Amazon DynamoDBを使ってみる(Management Console)
【AWS】2024年4月22日【AWS】API Gatewayを使ってみる
【AWS】2024年4月18日【AWS】Lambdaを使ってみる